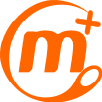 |
ManaPlus
|
Go to the documentation of this file.
22 #ifndef LISTENERS_BASELISTENER_HPP
23 #define LISTENERS_BASELISTENER_HPP
27 #define defineListener(name) \
28 STD_VECTOR<name*> name::mListeners; \
37 removeListener(this); \
40 void name::addListener(name *const listener) \
43 mListeners.push_back(listener); \
46 void name::removeListener(const name *const listener) \
48 STD_VECTOR<name*>::iterator it = mListeners.begin(); \
49 while (it != mListeners.end()) \
51 if (*it == listener) \
52 it = mListeners.erase(it); \
58 #define defineListenerHeader(name) \
64 static void addListener(name *const listener); \
66 static void removeListener(const name *const listener); \
69 static STD_VECTOR<name*> mListeners;