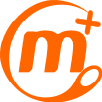 |
ManaPlus
|
Go to the documentation of this file.
22 #ifndef RENDER_OPENGL_MGLDEFINES_H
23 #define RENDER_OPENGL_MGLDEFINES_H
27 #ifndef GL_NUM_EXTENSIONS
28 #define GL_NUM_EXTENSIONS 0x821D
29 #define GL_DEPTH_ATTACHMENT 0x8D00
30 #define GL_COLOR_ATTACHMENT0 0x8CE0
31 #define GL_FRAMEBUFFER 0x8D40
32 #define GL_RENDERBUFFER 0x8D41
35 #ifndef GL_COMPRESSED_RGBA_ARB
36 #define GL_COMPRESSED_RGBA_ARB 0x84EE
37 #define GL_COMPRESSED_RGBA_S3TC_DXT5_EXT 0x83F3
38 #define GL_COMPRESSED_RGBA_FXT1_3DFX 0x86B1
40 #ifndef GL_MAX_ELEMENTS_VERTICES
41 #define GL_MAX_ELEMENTS_VERTICES 0x80E8
42 #define GL_MAX_ELEMENTS_INDICES 0x80E9
45 #ifndef GL_DEBUG_OUTPUT
46 #define GL_DEBUG_OUTPUT_SYNCHRONOUS 0x8242
47 #define GL_DEBUG_OUTPUT 0x92E0
48 #define GL_DEBUG_SOURCE_API 0x8246
49 #define GL_DEBUG_SOURCE_WINDOW_SYSTEM 0x8247
50 #define GL_DEBUG_SOURCE_SHADER_COMPILER 0x8248
51 #define GL_DEBUG_SOURCE_THIRD_PARTY 0x8249
52 #define GL_DEBUG_SOURCE_APPLICATION 0x824A
53 #define GL_DEBUG_SOURCE_OTHER 0x824B
54 #define GL_DEBUG_TYPE_ERROR 0x824C
55 #define GL_DEBUG_TYPE_DEPRECATED_BEHAVIOR 0x824D
56 #define GL_DEBUG_TYPE_UNDEFINED_BEHAVIOR 0x824E
57 #define GL_DEBUG_TYPE_PORTABILITY 0x824F
58 #define GL_DEBUG_TYPE_PERFORMANCE 0x8250
59 #define GL_DEBUG_TYPE_OTHER 0x8251
60 #define GL_DEBUG_TYPE_MARKER 0x8268
61 #define GL_DEBUG_SEVERITY_NOTIFICATION 0x826B
62 #define GL_DEBUG_SEVERITY_HIGH 0x9146
63 #define GL_DEBUG_SEVERITY_MEDIUM 0x9147
64 #define GL_DEBUG_SEVERITY_LOW 0x9148
67 #ifndef GL_DEBUG_TYPE_PUSH_GROUP
68 #define GL_DEBUG_TYPE_PUSH_GROUP 0x8269
70 #ifndef GL_DEBUG_TYPE_POP_GROUP
71 #define GL_DEBUG_TYPE_POP_GROUP 0x826a
74 #ifndef GL_EXT_debug_label
75 #define GL_PROGRAM_PIPELINE_OBJECT_EXT 0x8A4F
76 #define GL_PROGRAM_OBJECT_EXT 0x8B40
77 #define GL_SHADER_OBJECT_EXT 0x8B48
78 #define GL_BUFFER_OBJECT_EXT 0x9151
79 #define GL_QUERY_OBJECT_EXT 0x9153
80 #define GL_VERTEX_ARRAY_OBJECT_EXT 0x9154
83 #ifndef GL_ARRAY_BUFFER
84 #define GL_ARRAY_BUFFER 0x8892
85 #define GL_ELEMENT_ARRAY_BUFFER 0x8893
88 #ifndef GL_STREAM_DRAW
89 #define GL_STREAM_DRAW 0x88E0
90 #define GL_STATIC_DRAW 0x88E4
91 #define GL_DYNAMIC_DRAW 0x88E8
94 #ifndef GL_COMPILE_STATUS
95 #define GL_FRAGMENT_SHADER 0x8B30
96 #define GL_VERTEX_SHADER 0x8B31
97 #define GL_COMPILE_STATUS 0x8B81
98 #define GL_LINK_STATUS 0x8B82
99 #define GL_VALIDATE_STATUS 0x8B83
100 #define GL_INFO_LOG_LENGTH 0x8B84
103 #ifndef GL_DEPTH_CLAMP
104 #define GL_DEPTH_CLAMP 0x864F
105 #define GL_RASTERIZER_DISCARD 0x8C89
106 #define GL_SAMPLE_MASK 0x8E51
109 #ifndef GL_POLYGON_SMOOTH
110 #define GL_POLYGON_SMOOTH 0x0B41
113 #ifndef GL_DEPTH_BOUNDS_TEST_EXT
114 #define GL_DEPTH_BOUNDS_TEST_EXT 0x8890
117 #ifndef GL_TEXTURE_COMPRESSION_HINT_ARB
118 #define GL_TEXTURE_COMPRESSION_HINT_ARB 0x84EF
121 #ifndef GLX_CONTEXT_PROFILE_MASK_ARB
122 #define GLX_CONTEXT_PROFILE_MASK_ARB 0x9126
125 #ifndef GLX_CONTEXT_MAJOR_VERSION_ARB
126 #define GLX_CONTEXT_MAJOR_VERSION_ARB 0x2091
127 #define GLX_CONTEXT_MINOR_VERSION_ARB 0x2092
128 #define GLX_CONTEXT_FLAGS_ARB 0x2094
131 #ifndef GL_COMPRESSED_RGBA_BPTC_UNORM_ARB
132 #define GL_COMPRESSED_RGBA_BPTC_UNORM_ARB 0x8E8C