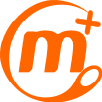 |
ManaPlus
|
Go to the documentation of this file.
42 #ifndef __has_extension
43 #define __has_extension(x) 0
46 #define __has_feature(x) 0
49 #define __has_include(x) 0
55 #if __cplusplus >= 201103L || \
56 defined(__GXX_EXPERIMENTAL_CXX0X__) || \
57 (defined(_MSC_VER) && _MSC_VER >= 1600)
58 #define NVWA_CXX11_MODE 1
60 #define NVWA_CXX11_MODE 0
66 #if !defined(HAVE_CXX11_ATOMIC)
67 #if NVWA_CXX11_MODE && \
68 ((__has_include(<atomic>) && !defined(__MINGW32__)) || \
69 (defined(_MSC_VER) && _MSC_VER >= 1700) || \
70 (((defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 405) || \
71 defined(__clang__)) && \
72 (!defined(__MINGW32__) || defined(_POSIX_THREADS))))
75 #define HAVE_CXX11_ATOMIC 1
77 #define HAVE_CXX11_ATOMIC 0
81 #if !defined(HAVE_CXX11_AUTO_TYPE)
82 #if NVWA_CXX11_MODE && \
83 (__has_feature(cxx_auto_type) || \
84 (defined(_MSC_VER) && _MSC_VER >= 1600) || \
85 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 404))
86 #define HAVE_CXX11_AUTO_TYPE 1
88 #define HAVE_CXX11_AUTO_TYPE 0
92 #if !defined(HAVE_CXX11_DELETED_FUNCTION)
93 #if NVWA_CXX11_MODE && \
94 (__has_feature(cxx_deleted_functions) || \
95 (defined(_MSC_VER) && _MSC_VER >= 1800) || \
96 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 404))
97 #define HAVE_CXX11_DELETED_FUNCTION 1
99 #define HAVE_CXX11_DELETED_FUNCTION 0
103 #if !defined(HAVE_CXX11_EXPLICIT_CONVERSION)
104 #if NVWA_CXX11_MODE && \
105 (__has_feature(cxx_explicit_conversions) || \
106 (defined(_MSC_VER) && _MSC_VER >= 1900) || \
107 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 405))
108 #define HAVE_CXX11_EXPLICIT_CONVERSION 1
110 #define HAVE_CXX11_EXPLICIT_CONVERSION 0
114 #if !defined(HAVE_CXX11_FINAL)
115 #if NVWA_CXX11_MODE && \
116 (__has_feature(cxx_override_control) || \
117 (defined(_MSC_VER) && _MSC_VER >= 1700) || \
118 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 407))
119 #define HAVE_CXX11_FINAL 1
121 #define HAVE_CXX11_FINAL 0
125 #if !defined(HAVE_CXX11_FUTURE)
126 #if NVWA_CXX11_MODE && \
127 ((__has_include(<future>) && !defined(__MINGW32__)) || \
128 (defined(_MSC_VER) && _MSC_VER >= 1700) || \
129 (((defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 405) || \
130 defined(__clang__)) && \
131 (!defined(__MINGW32__) || defined(_POSIX_THREADS))))
134 #define HAVE_CXX11_FUTURE 1
136 #define HAVE_CXX11_FUTURE 0
140 #if !defined(HAVE_CXX11_GENERALIZED_INITIALIZER)
141 #if NVWA_CXX11_MODE && \
142 (__has_feature(cxx_generalized_initializers) || \
143 (defined(_MSC_VER) && _MSC_VER >= 1800) || \
144 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 404))
145 #define HAVE_CXX11_GENERALIZED_INITIALIZER 1
147 #define HAVE_CXX11_GENERALIZED_INITIALIZER 0
151 #if !defined(HAVE_CXX11_LAMBDA)
152 #if NVWA_CXX11_MODE && \
153 (__has_feature(cxx_lambdas) || \
154 (defined(_MSC_VER) && _MSC_VER >= 1600) || \
155 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 405))
156 #define HAVE_CXX11_LAMBDA 1
158 #define HAVE_CXX11_LAMBDA 0
162 #if !defined(HAVE_CXX11_MUTEX)
163 #if NVWA_CXX11_MODE && \
164 ((__has_include(<mutex>) && !defined(__MINGW32__)) || \
165 (defined(_MSC_VER) && _MSC_VER >= 1700) || \
166 (((defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 403) || \
167 defined(__clang__)) && \
168 (!defined(__MINGW32__) || defined(_POSIX_THREADS))))
171 #define HAVE_CXX11_MUTEX 1
173 #define HAVE_CXX11_MUTEX 0
177 #if !defined(HAVE_CXX11_NOEXCEPT)
178 #if NVWA_CXX11_MODE && \
179 (__has_feature(cxx_noexcept) || \
180 (defined(_MSC_VER) && _MSC_VER >= 1900) || \
181 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 406))
182 #define HAVE_CXX11_NOEXCEPT 1
184 #define HAVE_CXX11_NOEXCEPT 0
188 #if !defined(HAVE_CXX11_NULLPTR)
189 #if NVWA_CXX11_MODE && \
190 (__has_feature(cxx_nullptr) || \
191 (defined(_MSC_VER) && _MSC_VER >= 1600) || \
192 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 406))
193 #define HAVE_CXX11_NULLPTR 1
195 #define HAVE_CXX11_NULLPTR 0
199 #if !defined(HAVE_CXX11_OVERRIDE)
200 #if NVWA_CXX11_MODE && \
201 (__has_feature(cxx_override_control) || \
202 (defined(_MSC_VER) && _MSC_VER >= 1600) || \
203 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 407))
204 #define HAVE_CXX11_OVERRIDE 1
206 #define HAVE_CXX11_OVERRIDE 0
210 #if !defined(HAVE_CXX11_RANGE_FOR)
211 #if NVWA_CXX11_MODE && \
212 (__has_feature(cxx_range_for) || \
213 (defined(_MSC_VER) && _MSC_VER >= 1700) || \
214 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 406))
215 #define HAVE_CXX11_RANGE_FOR 1
217 #define HAVE_CXX11_RANGE_FOR 0
221 #if !defined(HAVE_CXX11_RVALUE_REFERENCE)
222 #if NVWA_CXX11_MODE && \
223 (__has_feature(cxx_rvalue_references) || \
224 (defined(_MSC_VER) && _MSC_VER >= 1600) || \
225 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 405))
226 #define HAVE_CXX11_RVALUE_REFERENCE 1
228 #define HAVE_CXX11_RVALUE_REFERENCE 0
232 #if !defined(HAVE_CXX11_STATIC_ASSERT)
233 #if NVWA_CXX11_MODE && \
234 (__has_feature(cxx_static_assert) || \
235 (defined(_MSC_VER) && _MSC_VER >= 1600) || \
236 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 403))
237 #define HAVE_CXX11_STATIC_ASSERT 1
239 #define HAVE_CXX11_STATIC_ASSERT 0
243 #if !defined(HAVE_CXX11_THREAD)
244 #if NVWA_CXX11_MODE && \
245 ((__has_include(<thread>) && !defined(__MINGW32__)) || \
246 (defined(_MSC_VER) && _MSC_VER >= 1700) || \
247 (((defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 404) || \
248 defined(__clang__)) && \
249 (!defined(__MINGW32__) || defined(_POSIX_THREADS))))
252 #define HAVE_CXX11_THREAD 1
254 #define HAVE_CXX11_THREAD 0
258 #if !defined(HAVE_CXX11_THREAD_LOCAL)
259 #if NVWA_CXX11_MODE && \
260 (__has_feature(cxx_thread_local) || \
261 (defined(_MSC_VER) && _MSC_VER >= 1900) || \
262 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 408))
263 #define HAVE_CXX11_THREAD_LOCAL 1
265 #define HAVE_CXX11_THREAD_LOCAL 0
269 #if !defined(HAVE_CXX11_TYPE_TRAITS)
270 #if NVWA_CXX11_MODE && \
271 (__has_include(<type_traits>) || \
272 (defined(_MSC_VER) && _MSC_VER >= 1600) || \
273 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 403))
274 #define HAVE_CXX11_TYPE_TRAITS 1
276 #define HAVE_CXX11_TYPE_TRAITS 0
280 #if !defined(HAVE_CXX11_UNICODE_LITERAL)
281 #if NVWA_CXX11_MODE && \
282 (__has_feature(cxx_unicode_literals) || \
283 (defined(_MSC_VER) && _MSC_VER >= 1900) || \
284 (defined(__GNUC__) && __GNUC__ * 100 + __GNUC_MINOR__ >= 405))
285 #define HAVE_CXX11_UNICODE_LITERAL 1
287 #define HAVE_CXX11_UNICODE_LITERAL 0
294 #if HAVE_CXX11_DELETED_FUNCTION
295 #define _DELETED = delete
306 #if HAVE_CXX11_OVERRIDE
307 #define _OVERRIDE override
312 #if HAVE_CXX11_NOEXCEPT
313 #define _NOEXCEPT noexcept
314 #define _NOEXCEPT_(x) noexcept(x)
317 #define _NOEXCEPT throw ()
319 #define _NOEXCEPT throw()
321 #define _NOEXCEPT_(x)
324 #if HAVE_CXX11_NULLPTR
325 #define _NULLPTR nullptr
327 #define _NULLPTR NULL
330 #if HAVE_CXX11_THREAD_LOCAL
331 #define _THREAD_LOCAL thread_local
334 #define _THREAD_LOCAL __declspec(thread)
336 #define _THREAD_LOCAL __thread